Problem Statement
You are given an
The objective in this problem is to calculate the area of the region (T[0] xor T[1] xor ... xor T[W]). (See Notes for a formal definition.) The figures below show the region (T[0] xor T[1] xor ... xor T[W]) for W=1,2,3,4,5,6.
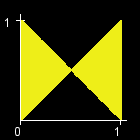
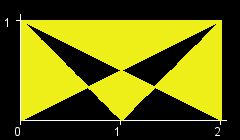
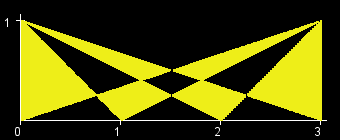
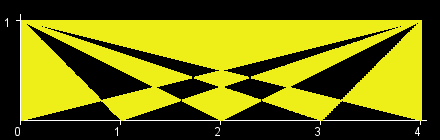
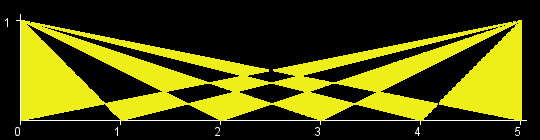
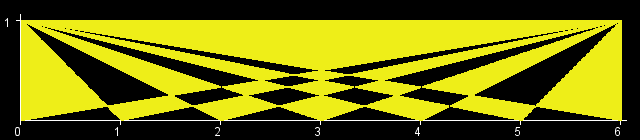
Return the integer part of the area of the region.
Definition
- Class:
- TriangleXor
- Method:
- theArea
- Parameters:
- int
- Returns:
- int
- Method signature:
- int theArea(int W)
- (be sure your method is public)
Notes
- For sets of points A and B in the XY-plane, the set (A xor B) is defined as the set of all points that lie in exactly one of the sets A and B (i.e., points that belong to the union of A and B but don't belong to their intersection).
- If the exact area is A, the correct return value is floor(A), not round(A). In words: you should return the largest integer that is less than or equal to the exact area.
- The format of the return value was chosen to help you in case of small precision errors. The constraints guarantee that computing the correct area with absolute error less than 0.01 is sufficient to determine the correct return value. The author's solution is significantly more precise than that.
Constraints
- W will be between 1 and 70,000, inclusive.
- The difference between the exact area of the region and the nearest integer will be greater than 0.01.
Examples
1
Returns: 0
The exact area is 0.5.
2
Returns: 1
The area is approximately 1.33333.
3
Returns: 1
The exact area is 1.35.
4
Returns: 2
The area is approximately 2.62857. Note that the correct answer is 2, not 3.
5
Returns: 2
The area is approximately 2.13294.
12345
Returns: 4629
6
Returns: 3
7
Returns: 2
8
Returns: 5
9
Returns: 3
10
Returns: 6
11
Returns: 4
12
Returns: 7
13
Returns: 5
126
Returns: 78
129
Returns: 48
247
Returns: 92
128
Returns: 80
192
Returns: 120
165
Returns: 62
181
Returns: 68
109
Returns: 41
239
Returns: 89
176
Returns: 110
62678
Returns: 39173
52183
Returns: 19568
54773
Returns: 20540
50991
Returns: 19121
61545
Returns: 23079
67741
Returns: 25403
55859
Returns: 20947
68053
Returns: 25520
57781
Returns: 21668
50892
Returns: 31807
70000
Returns: 43750
69999
Returns: 26249
69998
Returns: 43748
69997
Returns: 26249
69996
Returns: 43747
69995
Returns: 26248
69994
Returns: 43746
69993
Returns: 26247
9934
Returns: 6208
27635
Returns: 10363
37533
Returns: 14075
66823
Returns: 25058
46636
Returns: 29147
59791
Returns: 22421
20256
Returns: 12660
11160
Returns: 6975
44448
Returns: 27780
48882
Returns: 30551
38098
Returns: 23811
17056
Returns: 10660
63804
Returns: 39877
66666
Returns: 41666
29997
Returns: 11249
69977
Returns: 26241
1000
Returns: 625
56789
Returns: 21296